RESTful API: How It Works and What You Need to Know
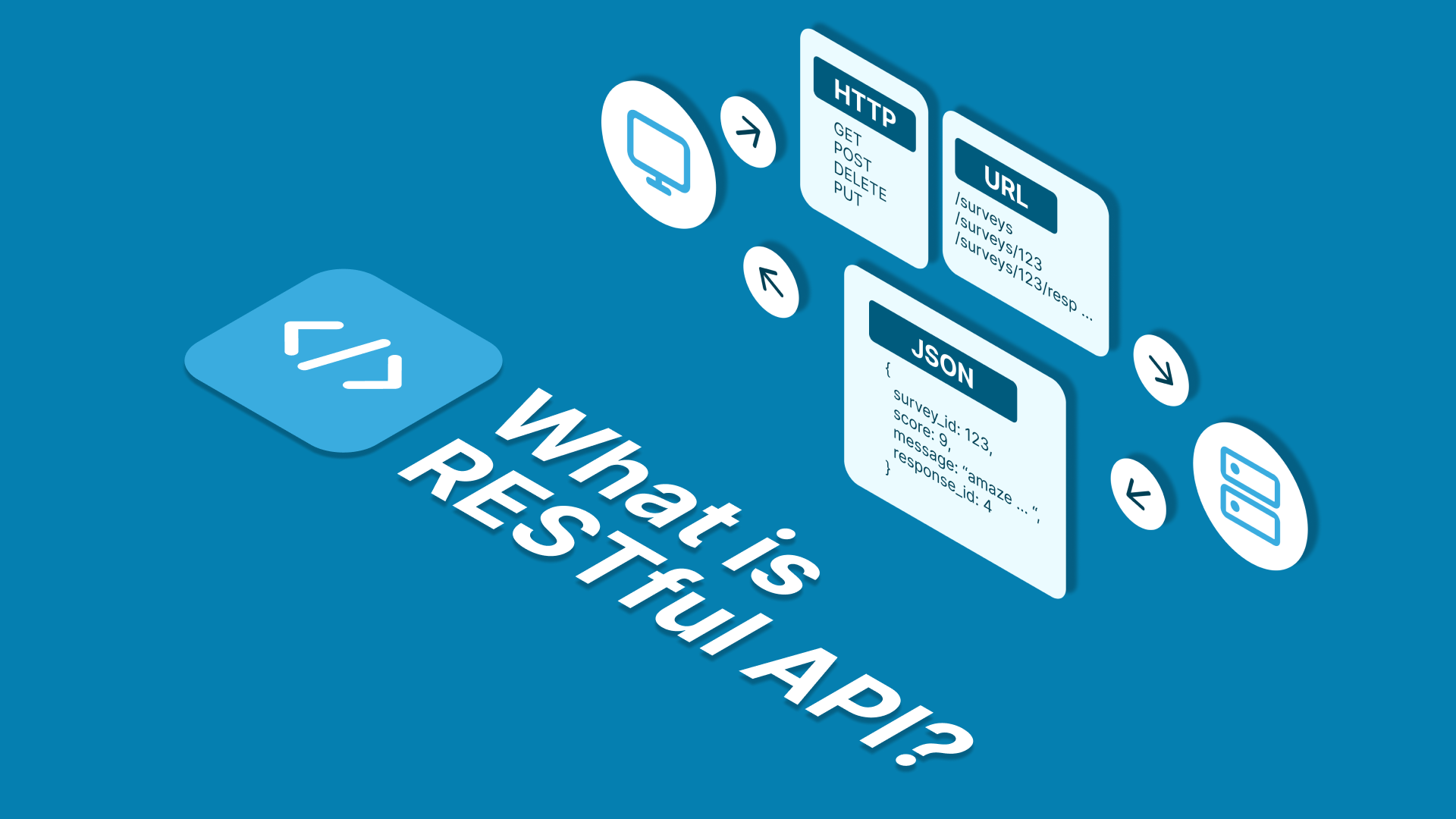
If you are a developer who wants to integrate your email system with other web applications, you might have heard of the term REST API. But what is REST API, and how does it work? In this article, we will explain the basics of REST API, its benefits, and how you can use it with our email services.
What is REST API?
REST stands for Representational State Transfer, and it is an architectural style for designing web APIs. A web API is an application programming interface (API) that allows communication between different web applications over the internet. For example, you can use a web API to access data from a social media platform, or to send an email from your website.
A REST API follows a set of architectural constraints that make it simple, scalable, and uniform. Some of these constraints are:
- Client-server: The client and the server are separate entities that communicate through requests and responses. The client initiates the request, and the server returns the response. This way, the client and the server can evolve independently without affecting each other.
- Stateless: The server does not store any information about the client's state. Each request from the client contains all the information that the server needs to process it. This makes the server more efficient and reliable.
- Uniform interface: The client and the server use a common language to exchange data. The uniform interface consists of four elements: resource identification, resource manipulation, self-descriptive messages, and hypermedia as the engine of application state (HATEOAS). We will explain these elements in more detail later.
- Layered system: The client and the server can interact through intermediate components, such as proxies or caches. These components can improve the performance and security of the system, but they do not affect the communication between the client and the server.
- Code on demand (optional): The server can send executable code to the client, such as JavaScript or Java applets. This can enhance the functionality of the client without changing its structure.
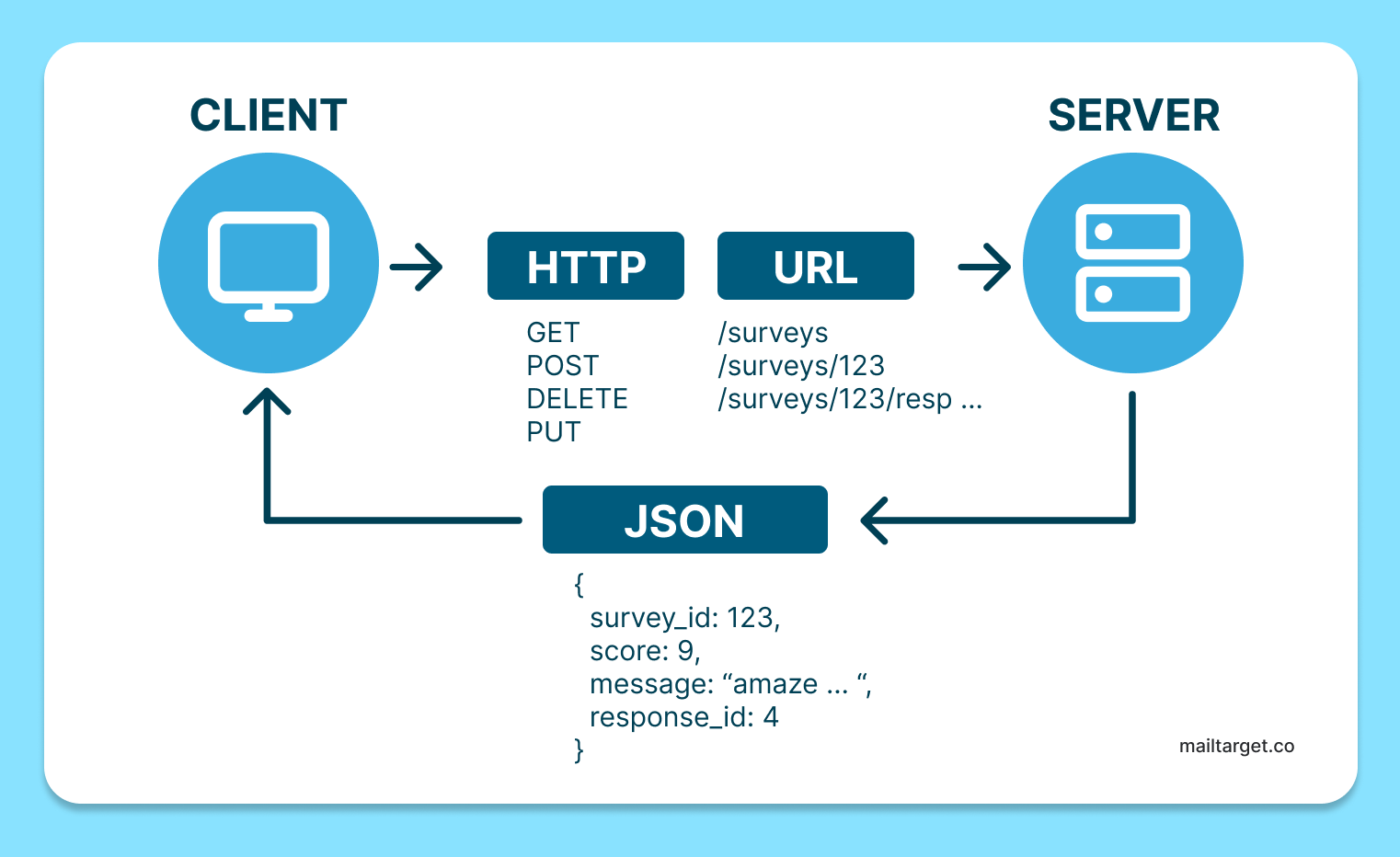
How RESTful API works?
To understand how RESTful API works, we need to understand some key concepts:
- Resource: A resource is anything that can be identified by a unique identifier, such as a URL. A resource can be a document, an image, a video, or any other type of data. For example, https://mailtarget.co/api/users is a resource that represents a collection of users.
- HTTP method: An HTTP method is a verb that indicates the action that the client wants to perform on a resource. The most common HTTP methods are GET, POST, PUT, PATCH, DELETE, and OPTIONS. For example, GET https://mailtarget.co/api/users returns a list of users, while POST https://mailtarget.co/api/users creates a new user.
- Representation: A representation is the format of the data that is exchanged between the client and the server. A representation can be in JSON (JavaScript Object Notation), XML (Extensible Markup Language), HTML (Hypertext Markup Language), or any other format that both parties can understand. For example, a JSON representation of a user might look like this:
{
"id": 1,
"name": "Alice",
"email": "[email protected]"
}
- Self-descriptive message: A self-descriptive message is a message that contains all the information that the receiver needs to understand it. A self-descriptive message includes headers and body. Headers provide metadata about the message, such as content type, content length, authorization, etc. Body contains the representation of the resource. For example, a self-descriptive message for creating a new user might look like this:
POST /api/users HTTP/1.1
Host: mailtarget.co
Content-Type: application/json
Content-Length: 55
Authorization: Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9...
{
"name": "Bob",
"email": "[email protected]"
}
- Hypermedia as the engine of application state (HATEOAS): HATEOAS is a principle that states that the response from the server should contain links to other related resources that the client can access. This way, the client can navigate through the application without knowing the exact URLs of the resources. For example, a response for getting a list of users might look like this:
{
"users": [
{
"id": 1,
"name": "Alice",
"email": "[email protected]",
"links": [
{
"rel": "self",
"href": "https://mailtarget.co/api/users/1"
},
{
"rel": "edit",
"href": "https://mailtarget.co/api/users/1/edit"
},
{
"rel": "delete",
"href": "https://mailtarget.co/api/users/1/delete"
}
]
},
{
"id": 2,
"name": "Bob",
"email": "[email protected]",
"links": [
{
"rel": "self",
"href": "https://mailtarget.co/api/users/2"
},
{
"rel": "edit",
"href": "https://mailtarget.co/api/users/2/edit"
},
{
"rel": "delete",
"href": "https://mailtarget.co/api/users/2/delete"
}
]
}
],
"links": [
{
"rel": "create",
"href": "https://mailtarget.co/api/users/create"
}
]
}
Why RESTful API is important?
RESTful API is important for web development because it offers many benefits, such as:
- Simplicity: RESTful API is easy to understand and implement, as it uses the existing features of the HTTP protocol. You do not need to learn any new syntax or tools to use REST API.
- Scalability: RESTful API can handle large volumes of data and requests, as it does not depend on the state of the server. You can easily add more servers or components to handle the load without affecting the existing ones.
- Performance: RESTful API can improve the performance of the system, as it allows caching and compression of the data. You can also use different representations for different devices or purposes, such as JSON for mobile apps or HTML for web browsers.
- Interoperability: RESTful API can work with any platform or language, as it uses standard formats and protocols. You can use REST API to communicate with any web application that supports HTTP and JSON, XML, or HTML.
How to use REST API with mailtarget?
You can use mailtarget’s REST API to send and receive emails, manage your contacts, campaigns, templates, and more. Some of the features of mailtarget’s REST API are:
- Email API: You can use the Email API to send transactional or marketing emails to your customers or users. You can customize your emails with dynamic content, attachments, images, etc. You can also track the delivery status, opens, clicks, bounces, and unsubscribes of your emails.
- SMTP Relay: You can use the SMTP Relay to send emails from your own application or server using SMTP protocol. You can use any SMTP client or library to connect to mailtarget’s SMTP server and send emails. You can also use mailtarget’s webhooks to receive notifications about your emails.
- Email SDK: You can use the Email SDK to integrate mailtarget’s email services with your preferred programming language or framework. mailtarget provides SDKs for PHP, Node.js, Python, Ruby, Java, and .NET. You can use the SDKs to send and receive emails, manage your contacts, campaigns, templates, and more.
mailtarget also provides a sandbox environment where you can test your email projects without affecting your production environment. You can use the sandbox environment to send test emails. You can switch to the production environment when you are ready to run your email projects live.
To use mailtarget’s REST API, you need to sign up for a free account and get your API key. You can use the API key to authenticate your requests to mailtarget’s REST API. You can also use the documentation to learn how to use mailtarget’s REST API with different languages and frameworks. Contact us for more information about how you can use mailtarget’s RESTful API.
(A.F)